Due to popular demand, I've added resolution independent scaling to Notrium. Previously if you played on a more modern monitor, the objects in the game might appear very small. This fix affects both the camera view of the game, and the inventory & journal screen.
The game view is scaled to how it used to look on my PC when I was developing the game back in 2003, so if you've only played the game recently, it may have appeared very different for you.
I'm ashamed to say, I saw the conversation when it started, and I thought they were just talking about the menu buttons. Then for some reason I just now read it again, and realized that I hadn't really understood what they were asking. 😳
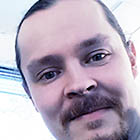
Something Is Stirring in the Kingdom… – Join Our Newsletter!
16.05.2025 by Ville MönkkönenView Comments
I’ve been working on new ideas, updates, and maybe even something big 👀! And the best way to stay in the loop? Our official newsletter. No spam, just the good stuff—development insights, sneak peeks, community highlights, and early access opportunities. Whether you've played for hours or just fell down the well at Northrop, you’re part of the story. And we want you along for what’s next.
If you don't want to hear from us very often, we've got you covered! I've just added more options to the Instant Kingdom newsletter. If you'd just like to know when our new game comes out, there's an option to receive a notice when we announce our new game, and when we release it. If you'd like to know a little more, there's an option to receive news of any major updates in addition to the announce/release news. And lastly, if you'd like to receive all of the updates to this blog, you can! So if you haven't already, sign up now!
I've converted everyone who signed up earlier to the Major Updates frequency, since that was the original wording of the newsletter form. But if you signed up through the game, you're now only at the lowest frequency (new releases and announcements), since that was the wording there. If you've already signed up earlier, you can change your preferred email frequency by typing your email on the same page.
I thought what would entice me to order the newsletter of a game developer I myself were interested in? The answer is obvious, a surprise🎁 would get me to sign up every time! So I'm promising everyone who signs up a (small) surprise. 😊
Hooray again! The Driftmoon Soundtrack is now available on Steam and GOG!
The official Driftmoon soundtrack, composed by the talented Gareth Meek. You won't need the Ooz slime earmuffs here! Includes lossless flac files. The music Gareth created gave the game a perfect blend of peace and excitement, and we couldn't imagine Driftmoon without it. We've unfortunately lost contact with our dear musician, so if you're reading this Gareth: Thank you!
Pro tip: There is a bundle to buy both the game and the soundtrack for a -20% price. If you already have the game, you won't need to buy a second copy, while you still get the -20% price for the soundtrack.
I love listening to music when I develop my games. My favourites are game soundtracks, especially melodic pieces from games of the 1990-2000 era. In case you're interested, here are some of the tracks I listen to: Ville's Favourite Game Music. Feel free to tip me off on any good additions to my list!
As it happens, there is also a free version of the Driftmoon soundtrack on YouTube, give it a go even if you're not planning on buying! This version doesn't get us any income however, so if you really find yourself listening to it more than a few times, or would like a better quality version, consider buying the soundtrack.
And to top it all, we've been sitting on some exciting news: Something new is brewing on the cooktop! (Yes, it's a new home-cooked, Instant Kingdom game! Yay!! 🤩) We can't reveal too much yet, but stay tuned for more. And do consider signing up to be notified when our new game is released. 😍
UPDATE: The soundtrack is now available on GOG too.
Hooray! I've updated the blog! I started this iteration of my blog in 2009 using Wordpress. It used to run on an Amazon SES instance. Because the instance was running a virtual Linux, it required constant updating from me, and so I moved the whole blog to Google App Engine in 2011, making my own version of Wordpress to run on the platform. They promised no need for me to maintain the server, and it was easy to code using Java and Eclipse, tools that I knew well. Since things rarely remain the same for long, it turned out that it did require some maintenance, constantly updating to new versions of libraries used by the Google backend. In the end their system became so bloated and difficult to understand, that I decided it was time to move on.
This time most of the user facing pages of my blog are good old static html. Being a programmer, I found it easiest to make my own templating engine. My blog posts live on my own PC, in .txt files, and are translated to .html files using my C# command line program. Then I upload all the changed files to my git repository, and the CloudFlare servers automatically update the files to their server. Simple and fast, the whole process takes only seconds! And it's free, so no complaints there!
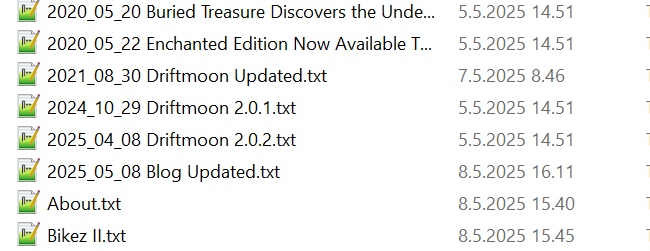
I've updated/repaired all of the old pages of the blog. You should go look at how Driftmoon started.
PS. I'm working on something new! Stay tuned for more news... And in case you haven't yet ordered it, sign up for our newsletter. We'll probably send it when our next game is out, so don't worry about it filling up your inbox.
- Made game window resizeable.
- Fixed bug in the crypt level where you could travel outside the playable area.
- Fixed message bottle and wizard hat size, they were ever so tiny!
- Mystery box server interface removed because of lack of use.
- Fixed companions going missing when quick traveling from the Nomon Docs warehouse, and other places.
- The little box companion is now unkillable.
- No longer possible to drop to lava from the end game fortress.
- Fix being able to quick travel out of the locked tavern at Northrop.
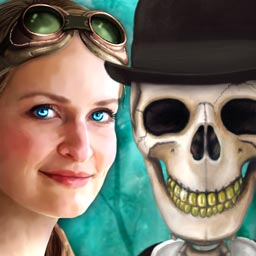
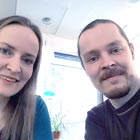
To celebrate our new icon, I've updated Driftmoon! New release 1.8.6 also brings some bugfixes:
- Driftmoon is now available on the Microsoft Store!
- Fixed mystery box bug.
- Fix the trapped fly escaping before the player met him near the end fortress.
- Fixed Bill sometimes dying from explosion near the end fortress.
- Fixed Mother Wolf sometimes getting killed before the player even met her.
- Various minor bugs and fixes.
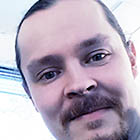
Enchanted Edition Now Available To Instantkingdom.com buyers
22.05.2020 by Ville MönkkönenView Comments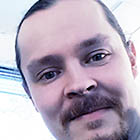
Buried Treasure Discovers the Underground Valuables of Driftmoon
20.05.2020 by Ville MönkkönenView Comments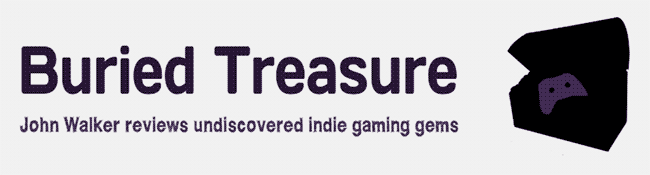

![]() | Get it on Google Play |
![]() | Download on the App Store |
![]() | Get Driftmoon on GOG.com |
![]() | Get Driftmoon on STEAM |
![]() | Get Driftmoon on HUMBLE BUNDLE |
![]() | Driftmoon demo for Windows |
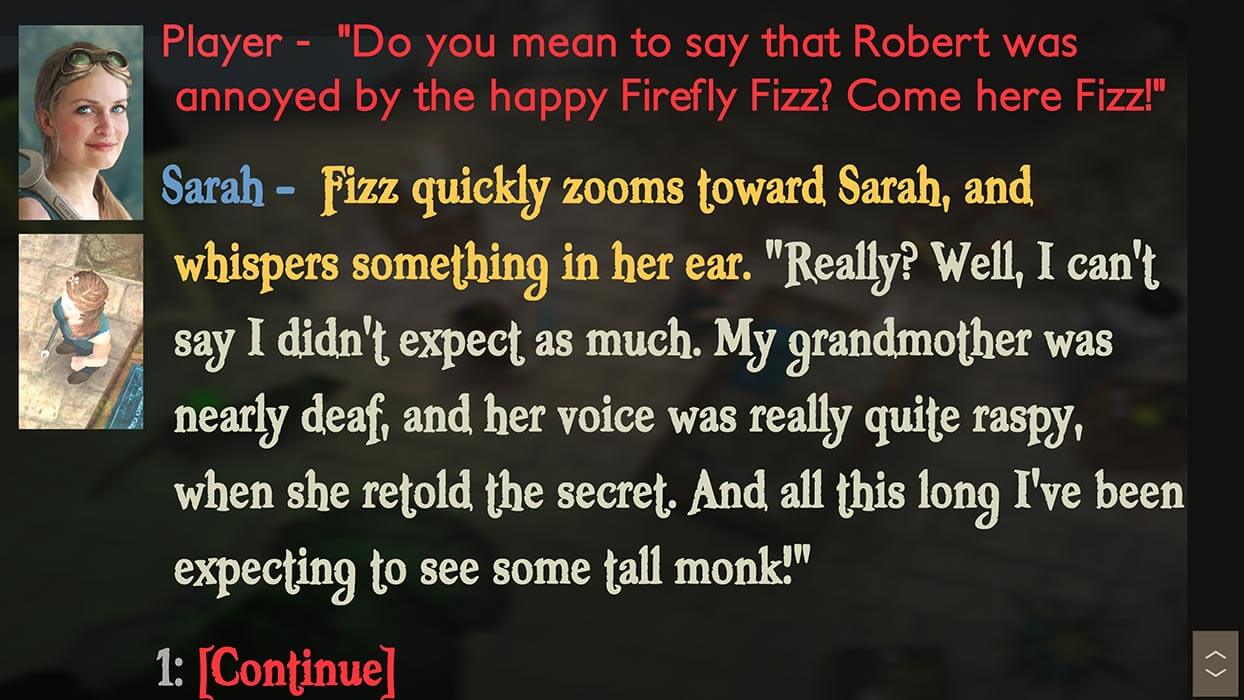
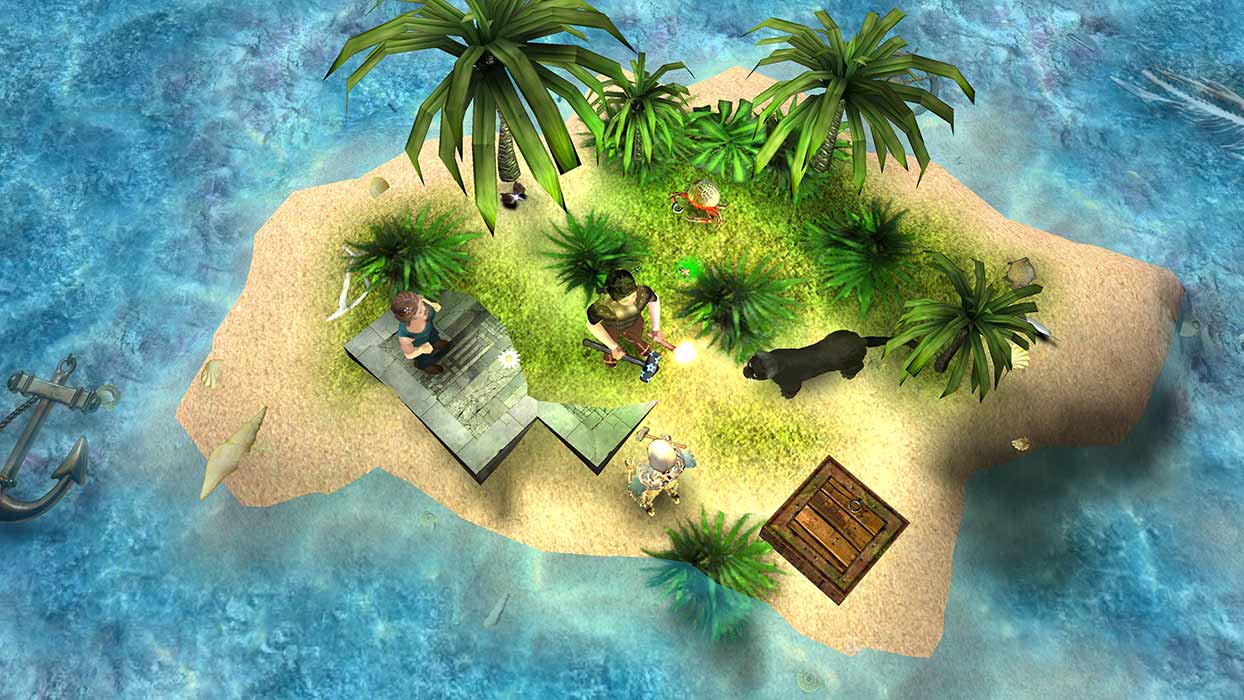
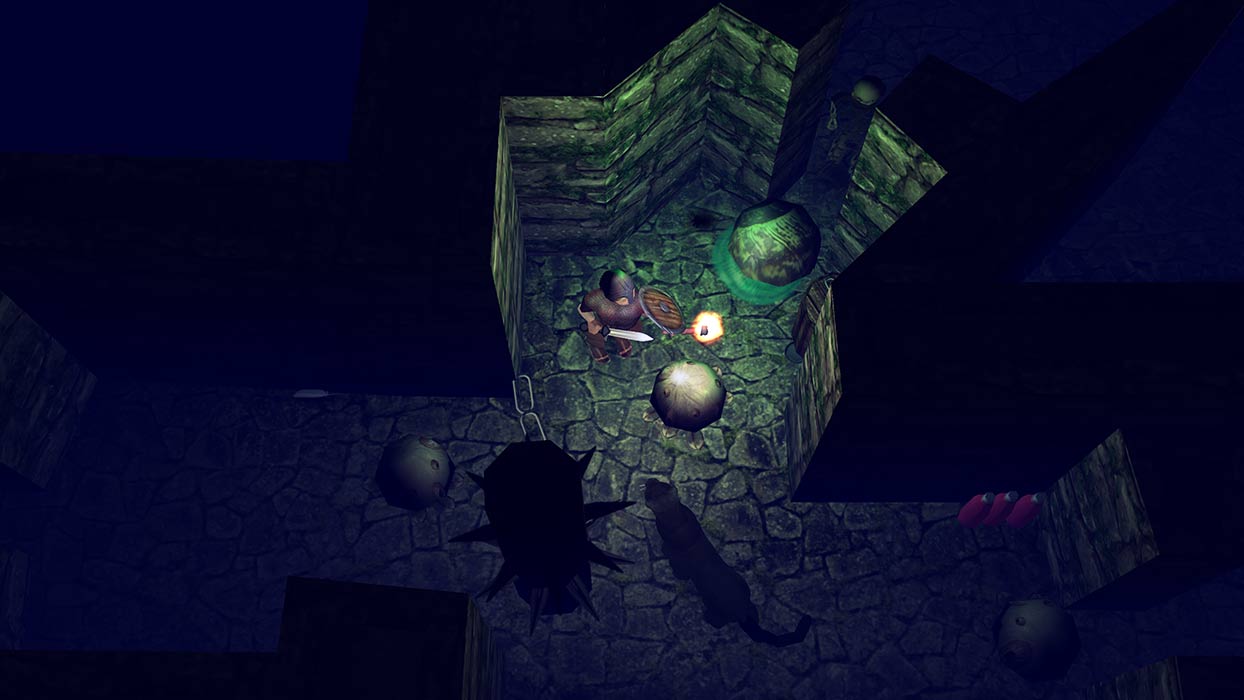
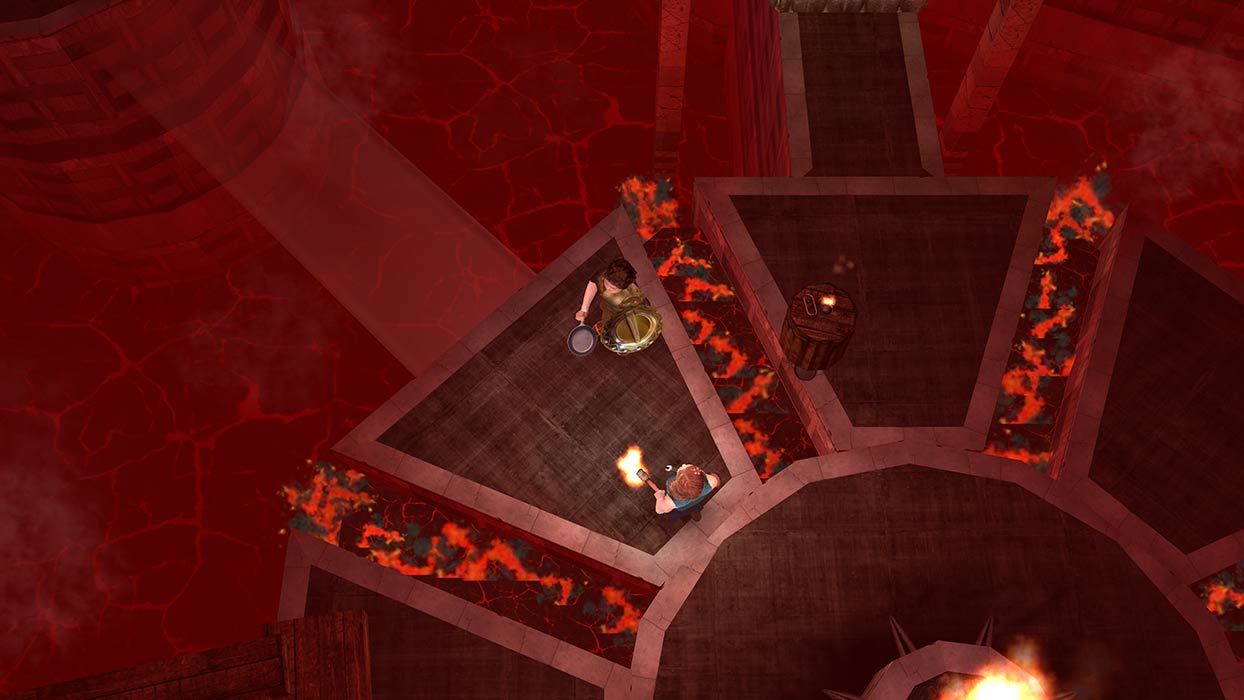
- Driftmoon now runs on Windows, Mac, Linux, iOS and Android - so take out your tablet or your cellphone and head straight for adventure!
- We've added the Mystery Box, full of surprises for both you and your friends!
- New characters, quests, items and skills (through the Mystery Box and elsewhere too)
- Major graphics update: better textures to look better on modern screens, lightmapped shadows, and the lot.
- Updated user interface: easier to use, looks better, and scales better: from your smallest screen to your living room TV. (still including the possibility to adjust text size, etc)
- Tons of bugfixes, dialogue fixes, quest fixes and improvements.
- Improved music, with an extra melody. All music by Gareth Meek - Thank you again, Gareth!
- Gameplay enhancements: quest reminders, Silver feather counter (very useful!), and more.
- The possibility to recover from a broken save (for example if your disk was full).
- And more surprises! Because there's bound to be something cool that we forgot to mention here.😆